Dynamic image resizing in Laravel
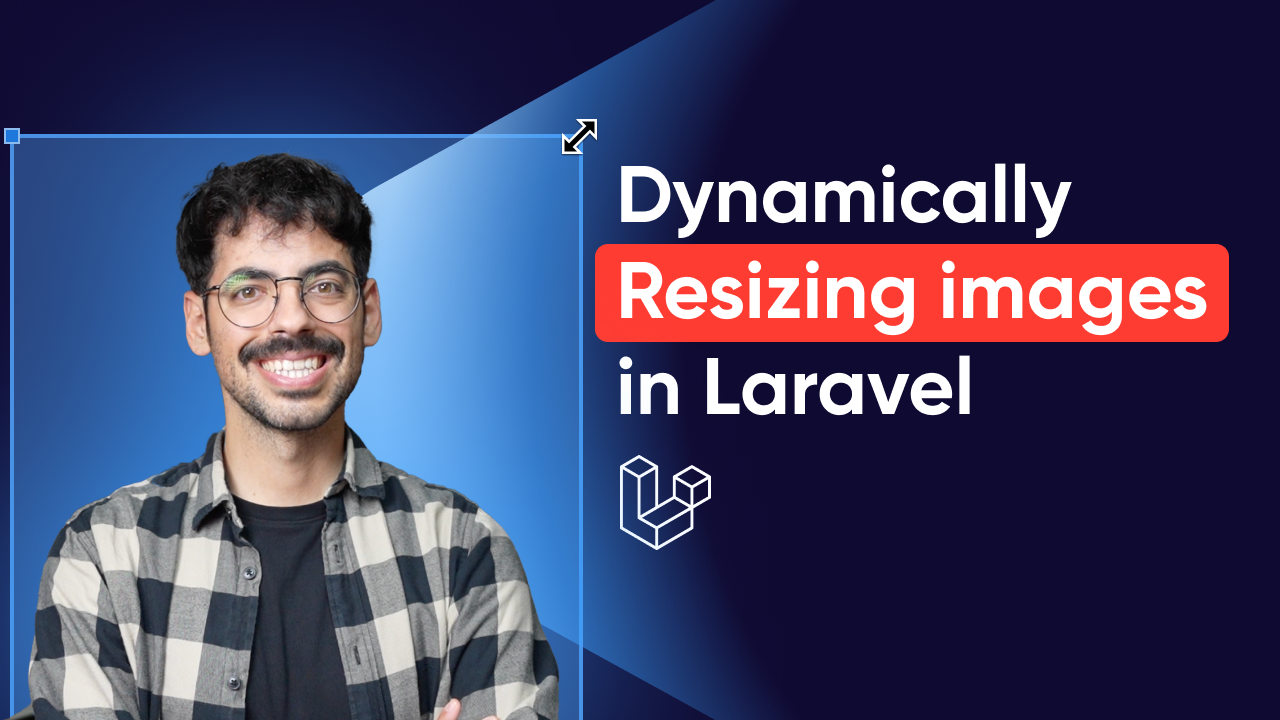
When it comes to resizing images in Laravel, every developer seems to have their own favorite way of doing it. Some developers prefer to use the Spatie medialibrary, others prefer to use commercial tools like Image Kit, Imgix or Cloudinary.
And then you have developers like me, who like to roll their own solution - which is exactly what I’ll be talking about today.
You can read the article below, or take a look at my Youtube video:
In my multitenant food ordering app, we have an infrastructure that looks a little something like this.
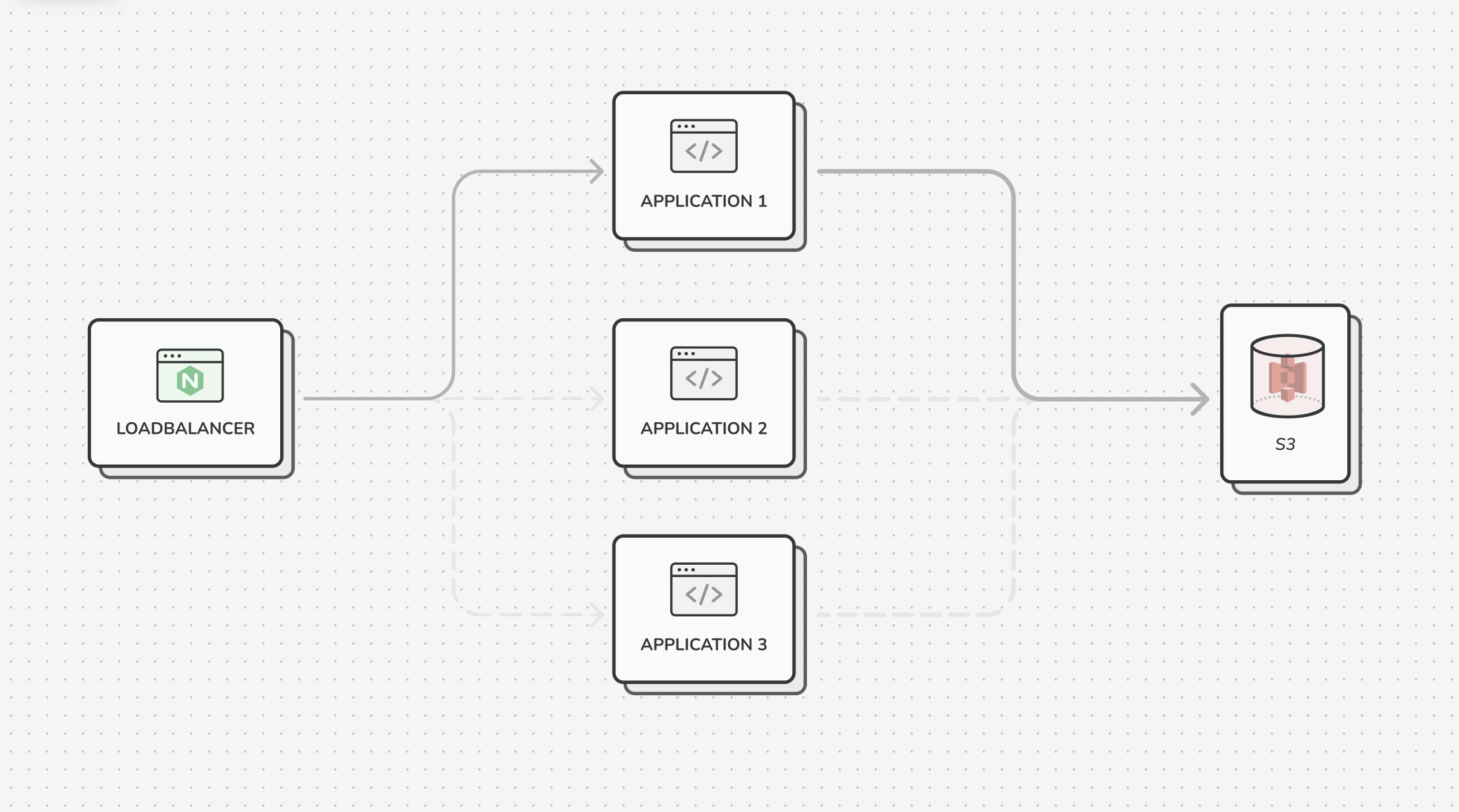
We have a loadbalancer with multiple application servers, and because we have multiple application servers, we use an S3 bucket to store our images.
We have a variety of frontends and each of them has a different need when it comes to displaying images, so we needed a solution that was flexible and most importantly, was able to dynamically resize images on the fly. We store tens of millions of images and we didn’t want to regenerate new images every time we needed a new format.
That’s where the AWS Dynamic Image Transformation template comes in handy. This template is freely available in the AWS solutions library, and you can deploy it with a few clicks.
The way solution works is pretty simple:
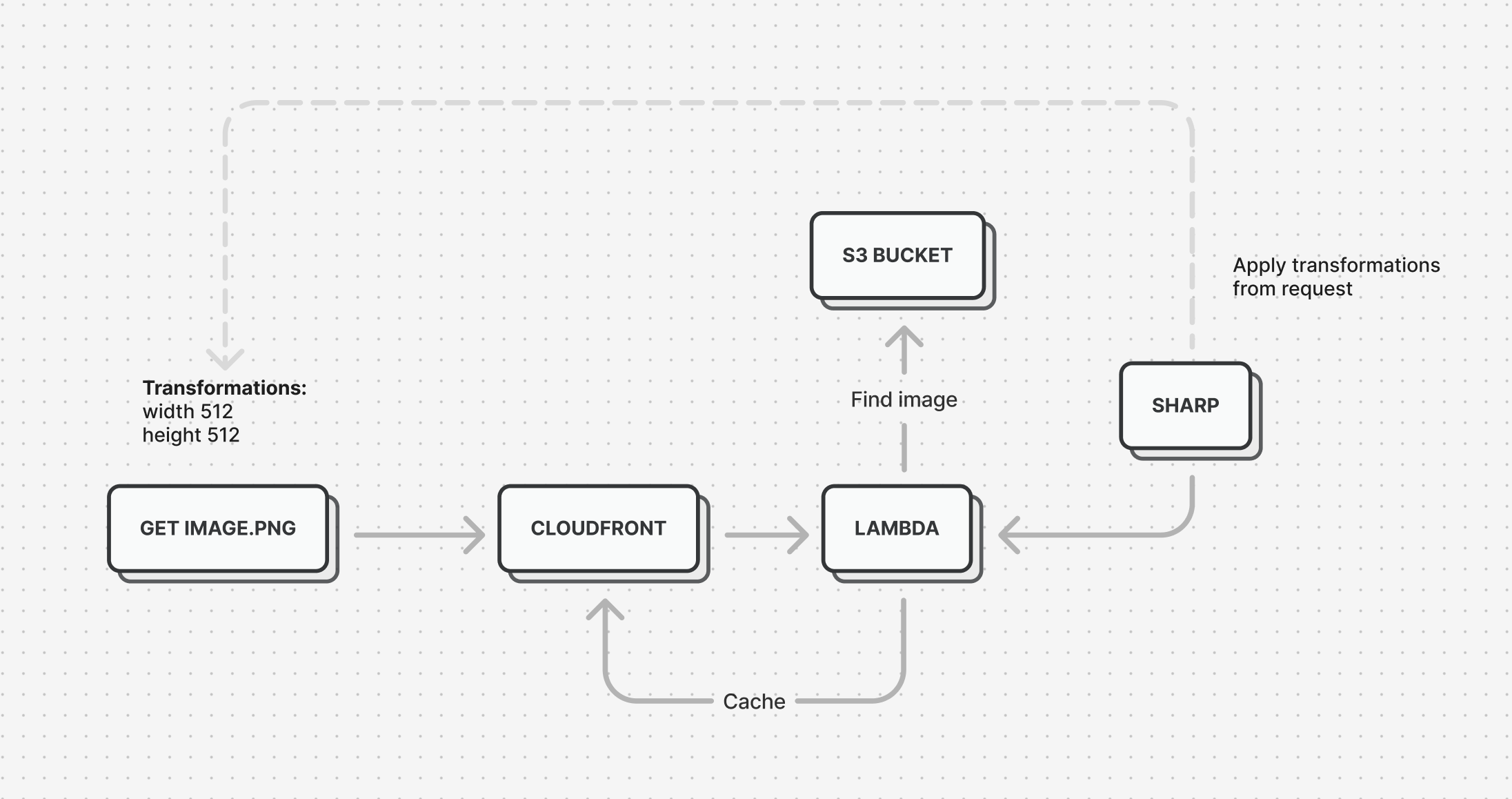
Whenever a request comes in, a function gets triggered that will look up the image in your S3 bucket, and in the request you can pass your image transformations that will get applied using a node library called Sharp. The resulting image gets cached in the CloudFront CDN so it is blazing fast. Most importantly - this is very cost efficient, so we can enjoy dynamically resized images at the fraction of the cost of other commercial solutions.
Deploying the AWS solution
You can deploy the solution by following the steps in the video linked above.
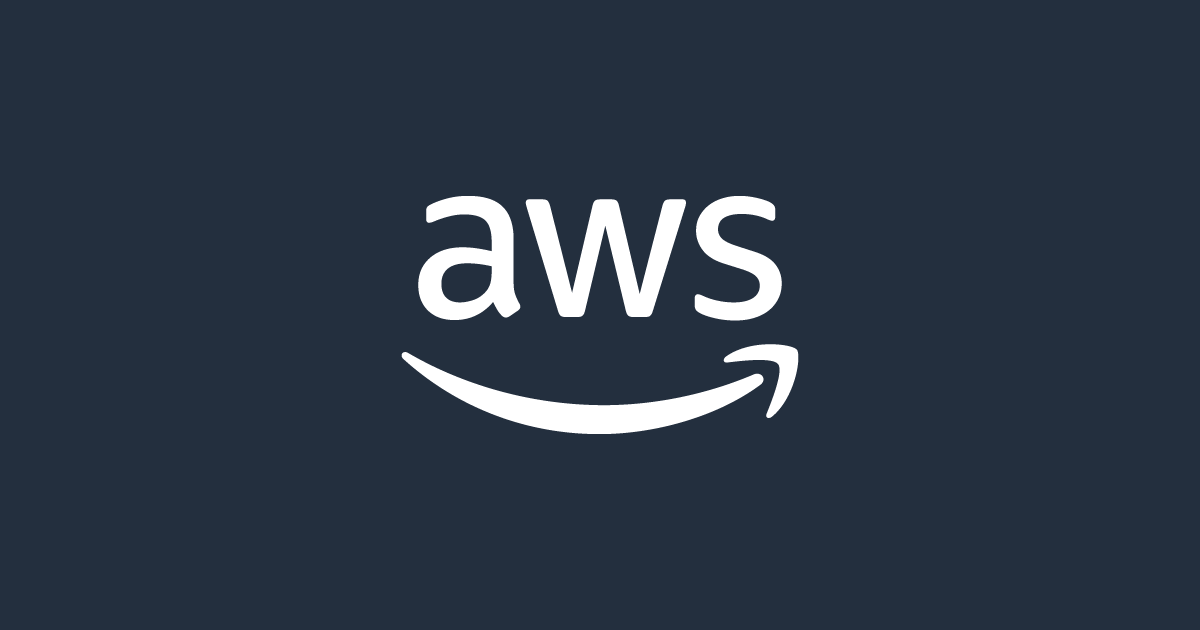
I created a small demo repository - it is very basic but it allows you to upload an image and do some basic transformations.
Let’s make sure our .env is filled out correctly:
AWS_ACCESS_KEY_ID=...
AWS_SECRET_ACCESS_KEY=...
AWS_DEFAULT_REGION=...
AWS_BUCKET=...
AWS_USE_PATH_STYLE_ENDPOINT=false
# Find this in the output of your CloudFormation template
CLOUDFRONT_URL=...
Then, upload an image and resize the image as to 512x512 - and if we take a look at the image now we’ll see it has been resized.
Let’s guide our attention to the URL - this is a base64 encoded string that contains our modifications, let’s decode the string:
eyJidWNrZXQiOiJ5b3V0dWJlLXNlcnZlcmxlc3MtaW1hZ2UtaGFuZGxlci1ldS1jZW50cmFsLTEiLCJrZXkiOiJpbWFnZXNcLzExXC9vcmlnaW5hbC5wbmciLCJlZGl0cyI6eyJyZXNpemUiOnsid2lkdGgiOiI1MTIiLCJoZWlnaHQiOiI1MTIiLCJmaXQiOiJjb3ZlciJ9fX0=
and here we’ll see the JSON payload that we send to the Lambda function.
{
"bucket": "youtube-serverless-image-handler-eu-central-1",
"key": "images\/11\/original.png",
"edits": {
"resize": {
"width": "512",
"height": "512",
"fit": "cover"
}
}
}
Now, you might think, how do we construct this payload? Well, I got you covered. If we dive into the ImagesController of our demo, we can see we use a Resizer class and this is a package I created you can simply pull into your project.
The Resizer has some convenient functions to set the dimensions of the image, the resize mode and a whole bunch of other modifications, like flipping the image, or applying a grayscale filter.
As you can see, this is pretty powerful stuff and I can’t stress the cost aspect enough, this is by far the cheapest solution I found to resize images dynamically, and I’ve been using it for many many years without any issues.
Hope this article was helpful, I'll see you in the next one! 👋
No spam, no sharing to third party. Only you and me.